This article comprises of some good coding techniques in Elixir. However, it is up to you to accept or not. Some may look familiar to you; please excuse me for wasting your time. But, that can be a revision plus.
For better understanding, I created some examples and code requirements just for this article based on my previous experiences of coding in Elixir.
If Condition & Value
Requirement
I have a string variable called status where its possible values are "True"
and "False"
. The binary strings. I have to return true for "True"
and false
for "False"
.
Don’t Do
new_status =
if status == "True" do
true
else
false
end
Do
new_status = status == "True"
I experienced this in another way. In a certain table of a certain column where value is either 0
or 1
. One of my co programmer need to update them to Boolean values by somehow processing the logic. Initially, he used if
macro to achieve this but refactored it later.
Testing Screenshot
Condition by Pattern Matching
Requirement
I need to find whether a map contains two keys of name
& age
.
As we know the Map
module contains a definition by name has_key?/2
which takes a map and key to check whether the key is present in the map or not.
In our case I need to check for two keys. So, I need to use &&
condition
Don’t Do
is_map_has_keys = Map.has_key(map, :name) && Map.has_key?(map, key2)
Do
is_map_has_keys = match?(%{name: _, age: _}, map)
The extra cheese is, we can also pattern match for values as well…
Testing Screenshot
Checking nil value in if condition
Some times we encounter a situation to check whether a value is nil
or not.
Don’t Do
feeling =
if bank_balance == nil do
" I am sad :("
else
"I am happy :)"
end
Do
feeling =
if bank_balance do
" I am happy :)"
else
"I am sad :("
end
Testing Screenshot
String Pattern Matching
Requirement
I need to take out the currency_value from the currency with a currency_symbol in a string. I know it is a weird example. I just want to convey the idea of pattern_matching
over the strings.
Don’t Do
currency = "$500"
[currency_value] = String.split(currency, "$", trim: true)
Do
"$"<> currency_value = currency
Testing Screenshot
Debug like a Pro-Programmer
When I was a newbie in Elixir, I used to prefer writing IO.inspect
than IEx.pry()
. Even if I want to debug three or more variables, I used to write as many IO.inspect()
.Though it does the job, it is not a recommended way.
Requirement
I need to check the input parameters passed to a function
Don’t Do
#Filename: server.iex defmodule Server do
def send_packet(host, port, packet) do
IO.inspect host, label: "host"
IO.inspect port, label: "port"
IO.inspect packet, label: "packet"
end
end
Do
defmodule Server do require IEx
def send_packet(host, port, packet) do
IEx.pry()
end
end
With above style of coding you can check all the previous variable values available in namespace.
Testing Screenshot
In Case Don’t match for Conditions
Always avoid matching Boolean values in case
. That’s make no sense of using them as they aren’t. Use cond
instead.
Requirement
Finding the role of the user.
Don’t Do
role =
case admin? do
true ->
"Admin"
false ->
case sub_admin? do
true ->
"Sub Admin"
false ->
"General User"
end
end
Do
role =
cond do
admin? ->
"Admin"
sub_admin? ->
"Sub Admin"
true ->
"General User"
end
Testing Screenshot
Safe side Condition Evaluation
Requirement
Finding whether a programmer has a girl_friend or not. Just kidding. Here, programmer is a map.
Consider there are two programmer maps by names programmer1
and programmer2'
programmer1 = %{has_girlfriend: true, lang: "Elixir", type: "functional"}
programmer2 = %{lang: "C", type: "structured"}
Don’t Do
iex> if programmer1.has_girlfriend, do: "Lucky Guy", else: "Unlucky"
"Lucky Guy"
iex> if programmer2.has_girlfriend, do: "Lucky Guy", else: "Unlucky"
(KeyError) key :has_girlfriend not found in: %{lang: "C", type: "structured"}
Here, we are using .
to access the key has_girlfriend
from the map. It gives you a KeyError if a key is not present in a map.
Do
iex> if programmer1[:has_girlfriend], do: "Lucky Guy", else: "Unlucky"
"Lucky Guy"
iex> if programmer2[:has_girlfriend], do: "Lucky Guy", else: "Unlucky"
"Unlucky"
Here, if the key is not present, it gives you nil
value.
It is just a safe side coding.
Testing Screenshot
Hope you liked it. Feel free to exchange your ideas here…
If you find any other better ways of coding, please do let us know through your comments and let others get benefited from those.
Thanks for reading.
Check out the GitHub repository on Killer Elixir Tips
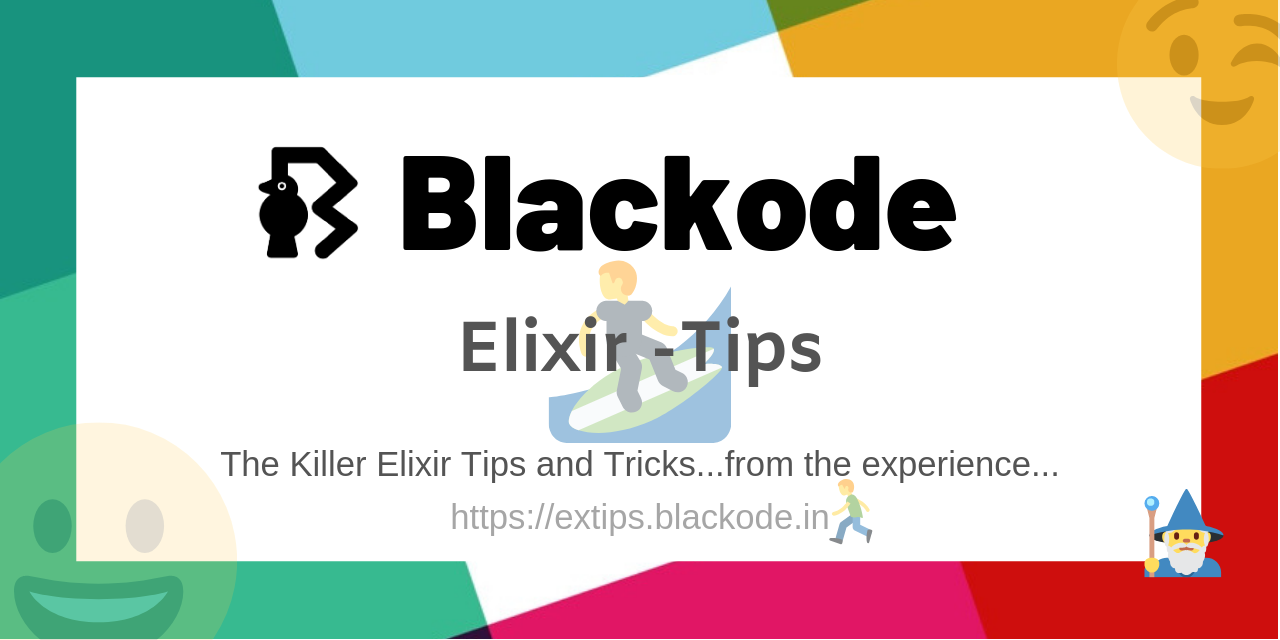
🎉 Happy Coding :)
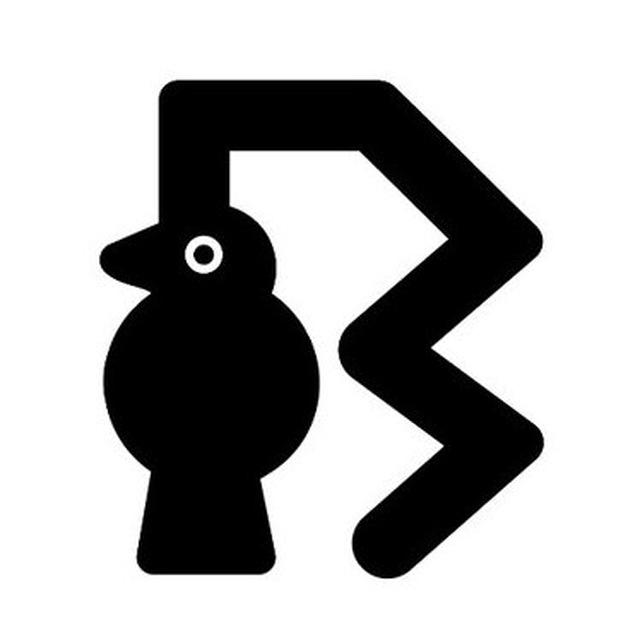